Beyond the Basics: Advanced Decoupling Strategies for Microservice Mastery
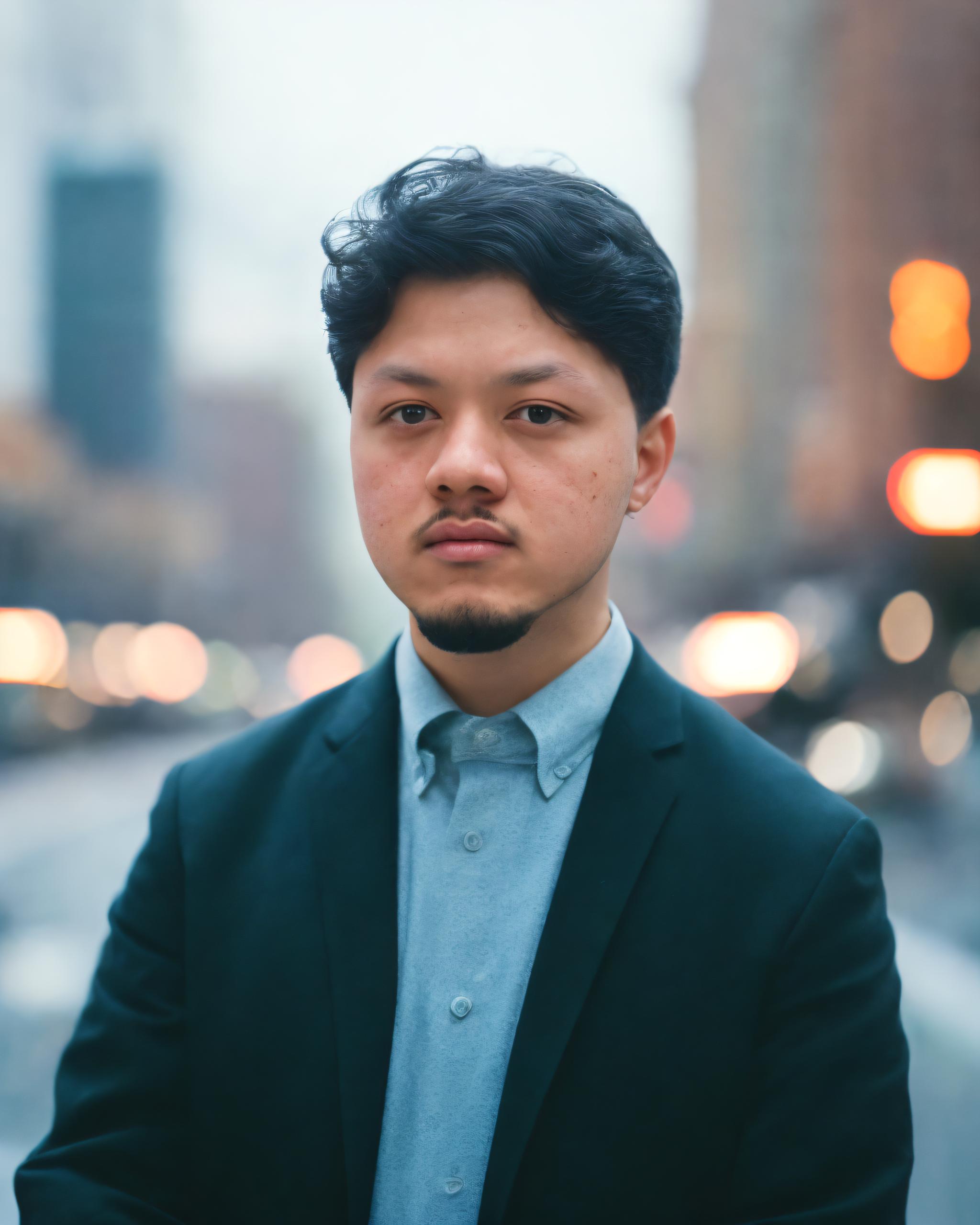
Beyond the Basics: Advanced Decoupling Strategies for Microservice Mastery
Last week I was talking to a really great developer and a friend of mine about a microservice architecture based project. We saw the issue of tight coupling between services (authentication service directly invoking the profile service for a database) and how it was challenging the very essence of microservice architecture. This led me to explore advanced decoupling strategies that can help architects and developers build resilient, scalable, and domain-aligned microservice architectures.
In this article, I will explore two advanced decoupling strategies: Event-Driven Architecture (EDA) enhanced with CQRS (Command Query Responsibility Segregation) and Domain Events, enriched with DDD (Domain-Driven Design) principles.
Understanding the Complexity: Tight Coupling in Microservices
In a typical microservice architecture, each service is expected to operate independently. However, dependencies, such as the need for the authentication service to directly invoke the profile service for database updates, introduce points of failure and complexity, challenging the very essence of microservice architecture.
Advanced Decoupling Techniques
To address these challenges, we propose two advanced strategies: Event-Driven Architecture (EDA) enhanced with CQRS ( Command Query Responsibility Segregation) and Domain Events, enriched with DDD (Domain-Driven Design) principles. These approaches are not just solutions but transformative strategies that encapsulate best practices for building resilient, scalable, and domain-aligned microservice architectures.
Strategy 1: EDA with CQRS for Operational Excellence
EDA facilitates communication between services through events, minimizing direct interactions. Integrating CQRS can further refine this by separating read and write operations, leading to more scalable and maintainable systems.
Implementing EDA with CQRS in Java: Quarkus and Spring Boot Insights
Quarkus Insight:
Quarkus can leverage EDA and CQRS (Command Query and responsibility Segregation) through its event-driven and reactive messaging capabilities. By separating commands (writes) from queries (reads), we ensure that complex operations like user registration and profile updates do not interfere with each other.
@ApplicationScoped
public class AccountService {
@Inject
@Channel("account-created")
Emitter<AccountCreatedEvent> accountCreatedEmitter;
@Transactional
public void createAccount(CreateAccountCommand command) {
// Account creation logic
accountCreatedEmitter.send(new AccountCreatedEvent(command.getAccountId()));
// ...
}
// Query methods here
}
With Spring Boot
Spring Boot, with Spring Cloud Stream and Spring Data, provides a rich set of functionalities to implement EDA alongside CQRS. This allows for a clean separation of concerns, enhancing system scalability and resilience.
@Service
public class AccountService {
private final StreamBridge streamBridge;
public AccountService(StreamBridge streamBridge) {
this.streamBridge = streamBridge;
}
@Transactional
public void createAccount(Command.CreateAccount command) {
// Account creation logic
streamBridge.send("accountCreatedChannel", new AccountCreatedEvent(command.getAccountId()));
// ...
}
// Query methods here
}
Strategy 2: Domain Events with DDD Principles
Domain Events signify meaningful domain-specific occurrences. Integrating these with DDD principles offers a nuanced approach to model complex business scenarios, enhancing the system’s expressiveness and resilience.
Leveraging Domain Events and DDD: A Senior Developer’s Approach
By encapsulating business logic into domain events and aligning them with DDD aggregates, entities, and value objects, we create a more cohesive and flexible architecture. This approach not only addresses decoupling but also enhances the domain model’s expressiveness and the system’s overall design quality.
public class UserRegisteredEvent extends DomainEvent {
private final UserId userId;
private final Email email;
public UserRegisteredEvent(UserId userId, Email email) {
this.userId = userId;
this.email = email;
// ...
}
// Getters for userId and email
}
Implementing these concepts requires a deep understanding of the domain; nonetheless, the benefits are substantial. By aligning the system with the domain, we create a more resilient and expressive architecture that can adapt to evolving business requirements.
Conclusion
These solutions are not just quick fixes but transformative strategies that encapsulate best practices for building resilient, scalable, and domain-aligned microservice architectures. By leveraging EDA with CQRS and Domain Events with DDD, architects and developers can address the complexities of microservice interactions and build systems that are truly aligned with the domain.
The journey of microservice architecture is really passionate and challenging. The advanced decoupling strategies explored here, EDA with CQRS and Domain Events with DDD, offer a way to address the complexities of microservice interactions. What would you choose? EDA with CQRS or Domain Events with DDD?
There are a lot of solutions to the problem of decoupling microservices, and the best one depends on the specific requirements and constraints of the system. However, the strategies discussed here are a great starting point for architects and developers looking to build resilient, scalable, and domain-aligned microservice architectures.
I am planning to write a series of articles on microservice architecture. If you have any specific topics in mind, feel free to share them with me. I would love to explore and write about them.
Even an e-book is in the pipeline. Stay tuned for more updates.